Archive for 2018
Write a program in C++ to find area of triangle
#include <iostream.h> #include <conio.h> int main() { float base, altitude; cout << "Base: "; cin >> base; cout << "Altitude: "; cin >> altitude; cout << endl << "Area:" << 0.5*base*altitude; getch(); return 0; }
Output:
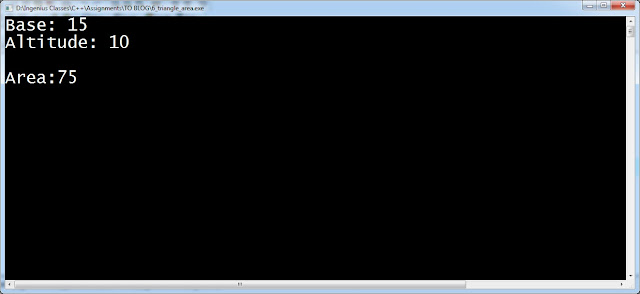
Write a program in C++ to find Highest of 3 numbers using nested if else
#include <iostream.h> #include <conio.h> int main() { int a, b, c; cout << "First No: "; cin >> a; cout << "Second No: "; cin >> b; cout << "Third No: "; cin >> c; if (a>b) { if(a>c) cout << a << " is highest"; else cout << c << " is highest"; } else { if(b>c) cout << b << " is highest"; else cout << c << " is highest"; } getch(); return 0; }
Output:
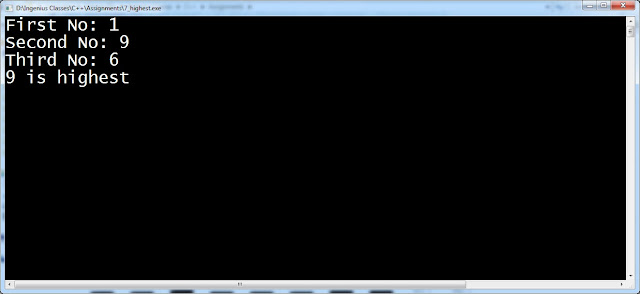
Search Tags: c++ programs, cpp programs, c++ practice programs, cpp practice programs, nested if else, highest number, maximum number
Write a program in C++ to print prime numbers from 1 to 100
#include <iostream.h> #include <conio.h> int main() { int count=0; for (int i=1; i<=100; i++) { for(int k=i; k>=1; k--) { if(i%k==0) { count++; } } if(count==1 || count==2) { cout << i << endl; } count=0; } getch(); return 0; }
Output:
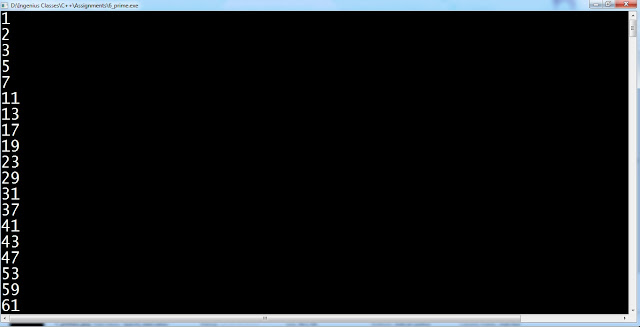
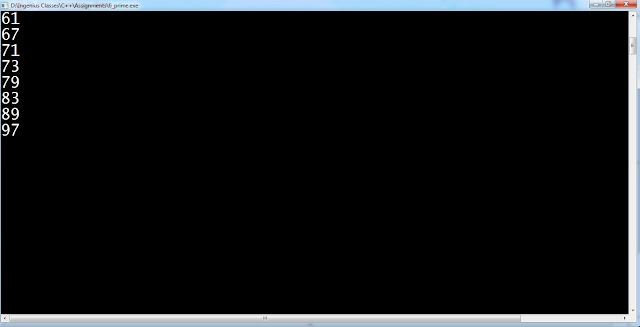
Search Tags: c++ programs, cpp programs, c++ practice programs, cpp practice programs, prime numbers
Write a program in C++ to find area of circle
#include <iostream.h> #include <conio.h> int main() { float r, area; cout << "Enter Radius:"; cin >> r; area = 3.14*r*r; cout << endl << "Area: " << area; getch(); return 0; }
Output:
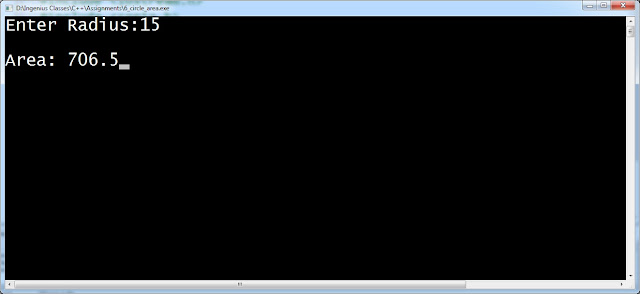
Write a program in C++ to check the number is positive or negative using Conditional Operator
#include <iostream.h> #include <conio.h> int main() { int a; cout << "Enter a:"; cin >> a; a>=0 ? cout<<"Positive" : cout<<"Negative"; getch(); return 0; }
Output:
Write a program in C++ to swap two variables without using third variable
#include <iostream.h> #include <conio.h> int main() { int a=10, b=15; a = a+b; b = a-b; a = a-b; cout << "a:" << a << endl; cout << "b:" << b << endl; getch(); return 0; }
Output:
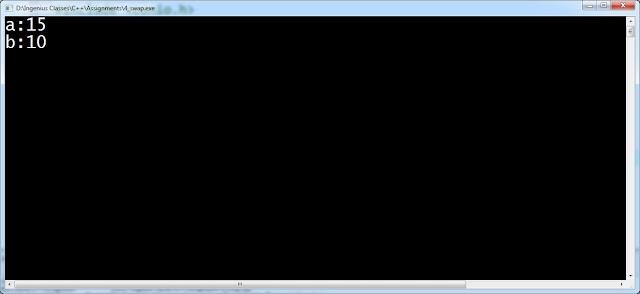
Write a program in C++ to find Addition, Subtraction, Multiplication, Division, Remainder and Average of two floating numbers
#include <iostream.h> #include <conio.h> int main() { float a, b; cout << "First No: "; cin >> a; cout << "Second No: "; cin >> b; cout << "Add:" << a+b << endl; cout << "Sub:" << a-b << endl; cout << "Div:" << a/b << endl; cout << "Mul:" << a*b << endl; cout << "Remainder:" << (int)a % (int)b << endl; cout << "Average:" << (a+b)/2 << endl; getch(); return 0; }
Output:
Write a program in C++ to input roll no, name and marks and display entered details and average marks
#include <iostream.h> #include <conio.h> int main() { int rollno; char name[30]; float mat, sci, com; cout << "Roll No: "; cin >> rollno; cout << "Name: "; cin >> name; cout << "\nEnter Marks:" << endl; cout << "Maths: "; cin >> mat; cout << "Science: "; cin >> sci; cout << "Computer: "; cin >> com; cout << "\n------\n\n"; cout << "Roll No: " << rollno << endl; cout << "Name: " << name << endl; cout << "Average: " << (mat+sci+com)/3; getch(); return 0; }
Output:
C++ Programs
Use CTRL+F to find the desired program
- Write a program in C++ to input roll no, name and marks and display entered details and average marks
- Write a program in C++ to find Addition, Subtraction, Multiplication, Division, Remainder and Average of two floating numbers
- Write a program in C++ to find the Square and Cube of any number
- Write a program in C++ to swap two variables without using third variable
- Write a program in C++ to check the number is positive or negative using Conditional Operator
- Write a program in C++ to find area of circle
- Write a program in C++ to find area of triangle
- Write a program in C++ to find simple interest
- Write a program in C++ to enter marks of 5 subjects and then calculate percentage
a. Percentage > 75 then print “Distinction”
b. Percentage > 60 print “First Class”
c. Percentage > 50 print “Second Class”
d. Percentage > 35 print “Pass”
e. Otherwise print “Fail” - Write a program in C++ to check whether the entered year is leap year or not
- Write a program in C++ to print prime numbers from 1 to 100
- Write a program in C++ to check whether the entered number if prime number or not
- Write a program in C++ to find Maximum of 3 number using Conditional Operator
- Write a program in C++ to find Maximum of 3 number using Nested If Else
- Write a program in C++ to find addition, subtraction, multiplication or division of 2 numbers entered by user using switch case
- Write a program in C++ to find out Area of Triangle, Rectangle or Circle using If else (Must be menu driven)
- Write a program in C++ to find factorial of entered number
- Write a program in C++ to print Fibonacci series upto number entered by user
- Write a program to print table of entered number
- Write a program to find max digit in entered number (e.g. in 1563 max digit is 6)
- Write a program to find summation of digits of any number (e.g. in 1563 summation is 1+5+6+3=14)
- Write a program to find summation of first and last digit of a number (e.g. in 1365 summation is 1+5=6)
- Write a program to print following pyramids
*
**
***
****1
12
123
12341
22
333
4444*
**
***
****1
21
321
4321
54321*
* *
* * *
* * * *
* * * * *1
1 2
1 2 3
1 2 3 4
1 2 3 4 51
2 2
3 3 3
4 4 4 4
5 5 5 5 5*
**
***
****
***
**
** * * * * *
* * * * *
* * * *
* * *
* *
** * * * * * * *
* * * * * *
* * * *
* *
*
* *
* * *
* * * *
* * *
* *
* - Write a program to enter 6 elements in array and print square of every element
- Write a program to enter 10 elements in array and print the number of even and odd elements in it
- Write a program to enter 10 elements in array and print summation and average of elements
- Write a program to print max of 10 elements of array
- Write a program to sort array in ascending order
- Write a program to sort array in descending order
- Write a program to find second smallest element of array
- Write a program to print Matrix using 2D array
- Write a program for addition and subtraction 2 Matrix using 2D array
- Write a program for multiplication of 2 Matrix using 2D array
- Write a program to find Max element in 2D array (Matrix)
- Write a program to convert string from uppercase to lowercase and vice versa
- Write a program to count the number of words without using string function
- Write a program to copy a string’s value into another without using string function
- Write a program to reverse the string without using string function
- Write a program to check whether the entered string is Palindrome or not
- Write a program to concatenate 2 strings without using string function
- Write a program to find addition, subtraction, multiplication and division of 2 numbers using Function
- Write a program to find square and cube of any number using Function
- Write a program to find factorial of any number using Function
- Write a program to print Fibonacci Series using Function
- Write a program to find min and max element of an array using Function
- Write a program to sort the array using Function
- Write a program to print the string in reverse order using Function
- Write a program to for factorial using Recursive Function
- Write a program to check whether the entered number if Palindrome or not using Recursive Function
- Write a program of Structure employee that provides following information: emp_no, emp_name, emp_age, emp_designation, emp_salary, emp_address
- Write a program of Structure employee that provides following information for 5 employees: emp_no, emp_name, emp_age, emp_designation, emp_salary, emp_address
- Write a program of structure student having roll_no and marks of 3 subjects of 5 students, then print the total marks and percentage of every student
- Write a program to make addition of 2 numbers using pointer
- Write a program to swap two variables using pointer
- Write a program to concatenate 2 strings using pointer
- Write a program to sort array using pointer and function
- Write a program to read data from file
- Write a program to write data into a file
- Write a program to append data into already existing file
- Write a program to calculate square of any number using Macro
- Write a program to assign any constant value (e.g. pi=3.14) to Macro and use it to find area of circle
UC Browser is NOT allowed to browser this website
UC Browser is restricted for this website.
Please use Google Chrome or any other browser to view the content of this website